Methods and Accessors in JavaScript objects By albro
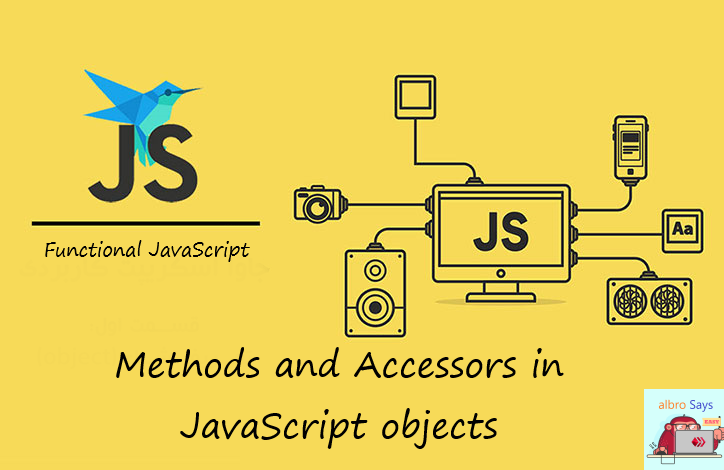
In this post, I'm going to talk about two different aspects of JavaScript objects: methods and accessors.
Object methods in JavaScript
Methods in JavaScript are actions that are performed on an object, but object method (that is, methods that belong to objects) is one of the properties of the object that has a function definition. To put it more simply, the methods of objects in JavaScript are the same functions that are placed inside objects to perform certain actions. See the following example:
var platform = {
firstName: "Hive",
lastName : "Blockchain",
id : 2020,
fullName : function() {
return this.firstName + " " + this.lastName;
}
};
In the fullName
attribute, we have the definition of a function. We call this function definition a method.
this
keyword
In the definition of a function, the this keyword refers to the owner of that function, so in the example above, this refers to the platform
object because the owner of the fullName
attribute is naturally that function. So it can be said that this.firstName
means the firstName
property of this object.
Access to object methods
To access the methods of an object, we use the following structure:
objectName.methodName()
Note: According to the example above, we call fullName()
a method and we call fullName
a property! Therefore, we have a property called fullName
, which is called by putting parentheses in front of it and executes the method inside it.
Let's look at some examples:
<!DOCTYPE html>
<html>
<body>
<p>Creating and using an object method.</p>
<p>A method is actually a function definition stored as a property value.</p>
<p id="demo"></p>
<script>
var platform = {
firstName: "Hive",
lastName : "Blockchain",
id : 2020,
fullName : function() {
return this.firstName + " " + this.lastName;
}
};
document.getElementById("demo").innerHTML = platform.fullName();
</script>
</body>
</html>
In this case, our output will be the definition of the function (method) itself!
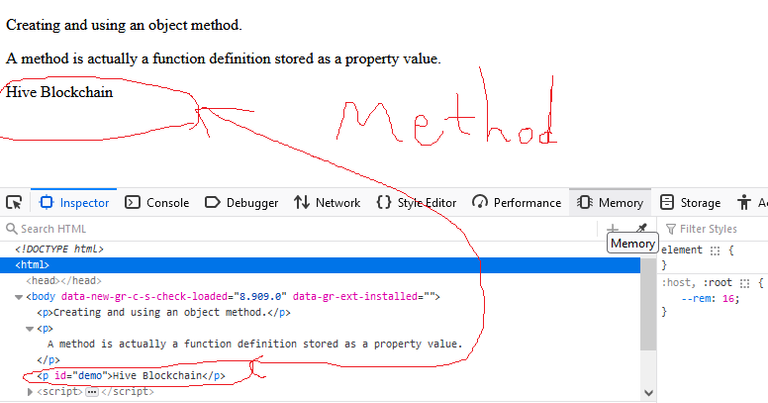
function() { return this.firstName + " " + this.lastName; }
Question: Can we add methods to an object?
Answer: Yes! This work is not difficult at all and is like adding properties to the object. The only difference is that you give it a function definition instead of a given value. Consider the following example:
<!DOCTYPE html>
<html>
<body>
<p id="demo"></p>
<script>
var platform = {
firstName: "Hive",
lastName : "Blockchain",
id : 2020,
};
platform.name = function() {
return this.firstName + " " + this.lastName;
};
document.getElementById("demo").innerHTML =
"My father is " + platform.name();
</script>
</body>
</html>
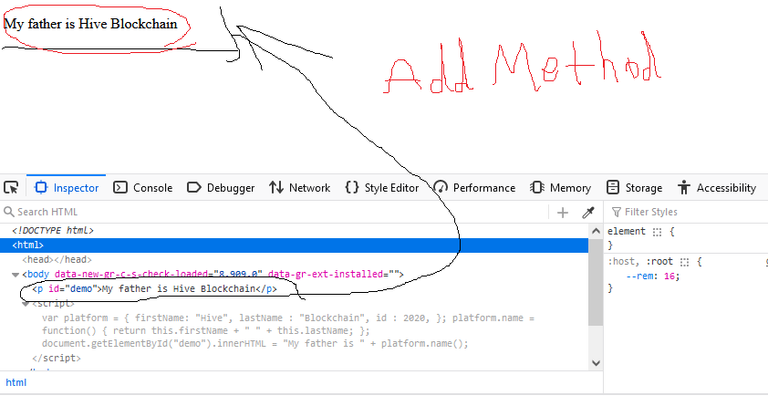
Prebuilt methods
In JavaScript, we have methods that have already been created by the developers of this language. I have given you an example of it so you can see how it works:
This example uses the toUpperCase()
method to convert a text string to an uppercase string:
var message = "Hello world!";
var x = message.toUpperCase();
By executing this statement, our initial string which is "Hello world!
" will become "HELLO WORLD!
".
Accessors in JavaScript
You may say to yourself, I'm fluent in JavaScript, but I don't know anything called Accessor! The reason is that few people use the word Accessor because it is a general term. Actually, we call Setters and Getters together as Accessors.
In fact, Accessors were introduced in 2009 with ECMAScript 5, and they allow you to access some properties of objects, and that's why they are called accessors (they give you access).
Get
keyword
To better understand this keyword, it is better to go directly to its examples.
In the following example, I use a property called lang
to get
the value of the language
property, that is, to receive:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Getters and Setters</h2>
<p>This example uses a lang property to get the value of the language property.</p>
<p id="demo"></p>
<script>
// Create an object:
var platform = {
firstName: "Hive",
lastName : "Blockchain",
language : "en",
get lang() {
return this.language;
}
};
// Display data from the object using a getter:
document.getElementById("demo").innerHTML = platform.lang;
</script>
</body>
</html>
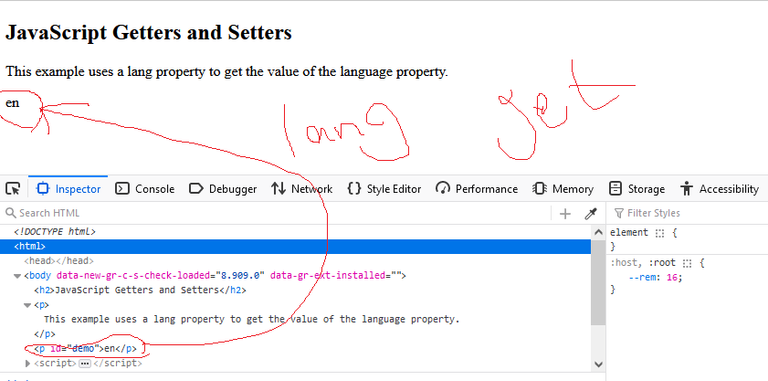
The output of this code will be en, but how? Let me explain to you step by step:
-
We have an object called
platform
. -
This object has properties (such as
firstName
,lastName
,language
, etc.). -
We create a property called
lang
(which is actually a function) using theget
keyword. -
lang
returns the value of thelanguage
attribute. -
Its output was
en
(short for English).
If we want to use setters in this example, we say:
var platform = {
firstName: "Hive",
lastName : "Blockchain",
language : "",
set lang(lang) {
this.language = lang;
}
};
// Specifies a property for the object
platform.lang = "en";
// Retrieves and displays data from the object
document.getElementById("demo").innerHTML = platform.language;
Yes, you guessed it right, using the lang
attribute, we have set the language
value. In this case, the output will be en
.
The difference between accessors and methods
What do you think is the difference between the two examples below?
var platform = {
firstName: "Hive",
lastName : "Blockchain",
fullName : function() {
return this.firstName + " " + this.lastName;
}
};
document.getElementById("demo").innerHTML = platform.fullName();
var platform = {
firstName: "Hive",
lastName : "Blockchain",
get fullName() {
return this.firstName + " " + this.lastName;
}
};
document.getElementById("demo").innerHTML = platform.fullName;
We access the fullName
property in both examples, but in the first example this is done through a function (platform.fullName()
) and in the second example through a property (platform.fullName
). There is no difference between these two methods, except that writing the codes in the second method is a little easier.
Note: You can also use method chaining in these examples. Pay attention to the following example:
var platform = {
firstName: "Hive",
lastName : "Blockchain",
language : "en",
get lang() {
return this.language.toUpperCase();
}
};
document.getElementById("demo").innerHTML = platform.lang;
In this example, I have used the toUpperCase
method when defining lang
, so its output will always be in capital letters, and we don't need to apply this statement to the output every time.
This is no different for setters:
set lang(lang) {
this.language = lang.toUpperCase();
}
Note: You may have heard of the Object.defineProperty()
statement. This statement can create the setters or getters you want. Pay attention to the following example:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Getters and Setters</h2>
<p>Perfect for creating counters:</p>
<p id="demo"></p>
<script>
// Define the initial object
var obj = {counter : 0};
// Define Setters and Getters
Object.defineProperty(obj, "reset", {
get : function () {this.counter = 0;}
});
Object.defineProperty(obj, "increment", {
get : function () {this.counter++;}
});
Object.defineProperty(obj, "decrement", {
get : function () {this.counter--;}
});
Object.defineProperty(obj, "add", {
set : function (value) {this.counter += value;}
});
Object.defineProperty(obj, "subtract", {
set : function (value) {this.counter -= value;}
});
// Testing codes
obj.reset;
obj.add = 5;
obj.subtract = 1;
obj.increment;
obj.decrement;
document.getElementById("demo").innerHTML = obj.counter;
</script>
</body>
</html>
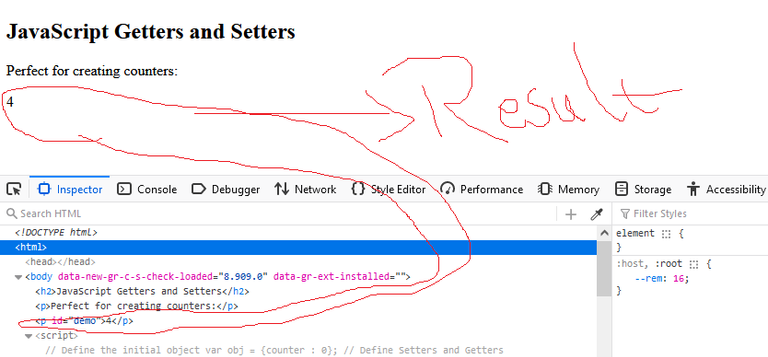
In the example, it is clear that this statement does not do anything special, but is another way to define setters and getters.
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🥇 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.