JavaScript Functions & Tricks By albro
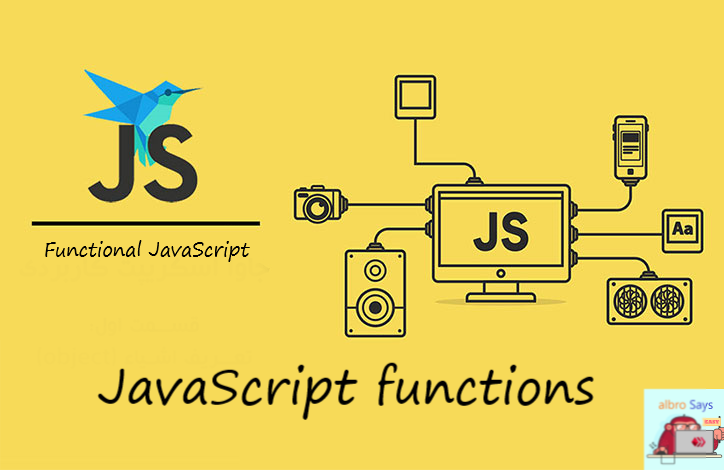
In this post, I want to talk about the types of functions (Self-invoking functions, Declaration functions, Expression functions, Arrow functions, etc.) and how to define them, and we will also give some points about these functions.
JavaScript functions
Functions in JavaScript are defined with the function
keyword and have two main types:
- Declaration Function
- Expression Function
Declaration Function
Functions are declared with the following structure:
function functionName(parameters) {
// The code you want to run in the function
}
These functions are not executed automatically, but we have to call them or invoke them in a more casual way. Their nature is such that they are stored for future use. Example:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Functions</h2>
<p>This example contains a statement that calls a function and then performs a calculation and returns a value. This function is of the first type (declarative):</p>
<p id="demo"></p>
<script>
var x = myFunction(4, 3);
document.getElementById("demo").innerHTML = x;
function myFunction(a, b) {
return a * b;
}
</script>
</body>
</html>
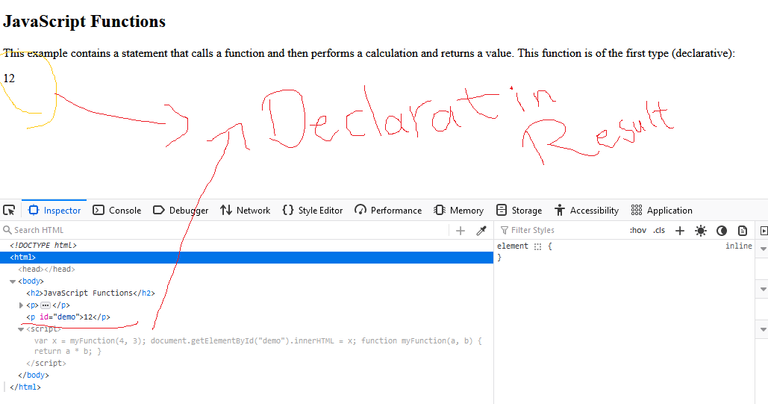
Note: semicolons are used to separate executable commands in JavaScript, and since declaring is not an executable function (I said that these types of functions are not executed at the same time until they are called), there is no need to put There is no semicolon in their definition (after the closing bracket).
Expression Function
Another way to define JavaScript functions is to define them through expression. These types of functions are stored in a variable:
<!DOCTYPE html>
<html>
<body>
<p>Storing functions of the second type inside a variable:</p>
<p id="demo"></p>
<script>
var x = function (a, b) {return a * b};
document.getElementById("demo").innerHTML = x;
</script>
</body>
</html>
After you define this type of function in a variable, you can use that variable as a function. The following example clearly shows this:
<!DOCTYPE html>
<html>
<body>
<p>After you define this type of function in a variable, you can use that variable as a function:
</p>
<p id="demo"></p>
<script>
var x = function (a, b) {return a * b};
document.getElementById("demo").innerHTML = x(4, 3);
</script>
</body>
</html>
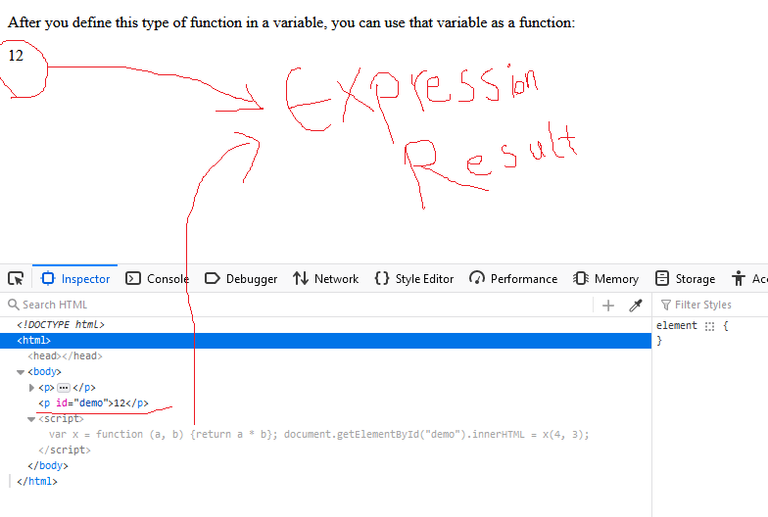
The function that you see in the above example is called anonymous function because it has no name. These types of functions are always called with the desired variable name.
Note: If you look carefully, I have used a semicolon in the above function because it is part of an executive statement.
Function() Constructor
You have seen from the previous examples that JavaScript functions are defined with the function
keyword, but there is another way to define functions in JavaScript. We can use a constructor: Function()
Consider the following example:
<!DOCTYPE html>
<html>
<body>
<p>JavaScript has an built-in function constructor.</p>
<p id="demo"></p>
<script>
var myFunction = new Function("a", "b", "return a * b");
document.getElementById("demo").innerHTML = myFunction(4, 3);
</script>
</body>
</html>
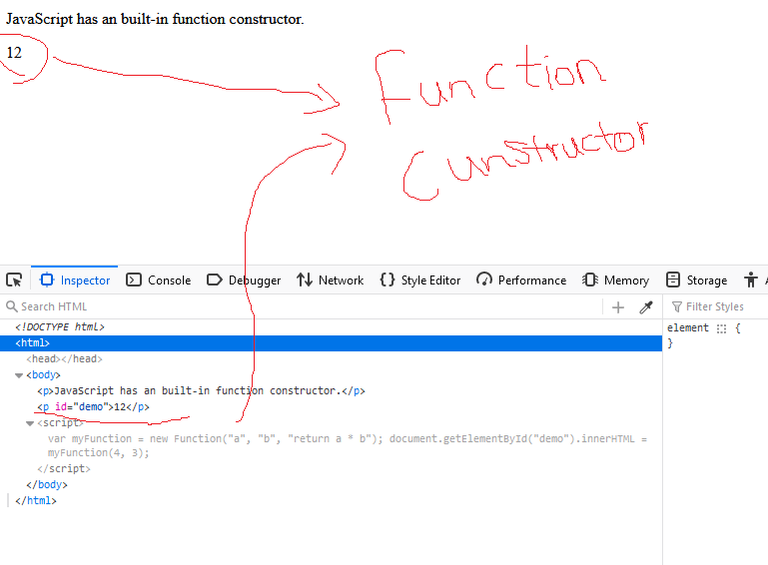
Do we have special functions that we need to use the constructor to define? No! This is just one of several methods of defining JavaScript functions. You can simply write the previous example as follows:
<!DOCTYPE html>
<html>
<body>
<p id="demo"></p>
<script>
var myFunction = function (a, b) {return a * b};
document.getElementById("demo").innerHTML = myFunction(4, 3);
</script>
</body>
</html>
Hoisting in JavaScript functions
JavaScript's default behavior is to move declarations to the top of the current scope. This is true for variables and functions, so we can call JavaScript functions before we define them:
myFunction(5);
function myFunction(y) {
return y * y;
}
But if we define the functions with expressions, they will no longer be hoisted and we cannot do this.
Self-invoking functions
Self-invoking functions means that they call themselves and do not need to be called and called separately. You can Self-invoking them by placing parentheses in front of Function expressions.
Note: function declaration cannot be Self-invoking.
Consider the following example:
<!DOCTYPE html>
<html>
<body>
<p>Functions can be invoked automatically without being called:</p>
<p id="demo"></p>
<script>
(function () {
document.getElementById("demo").innerHTML = "Hello! I called myself";
})();
</script>
</body>
</html>
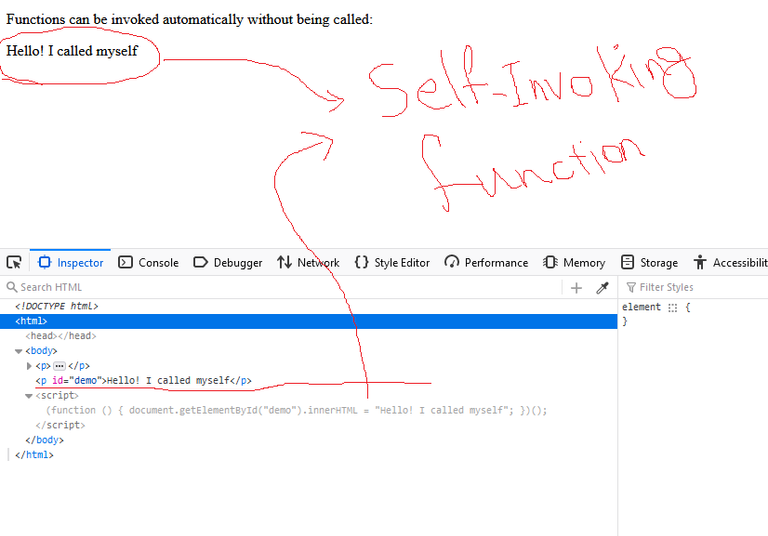
As you can see, in order to read the functions, you must first put the entire function in a pair of parentheses and then put another pair of parentheses in front of it!
It is interesting to know that the above example function is called anonymous self-invoking function.
Note: You can use functions as a single value:
<!DOCTYPE html>
<html>
<body>
<p id="demo"></p>
<script>
function myFunction(a, b) {
return a * b;
}
var x = myFunction(4, 3) * 2;
document.getElementById("demo").innerHTML = x;
</script>
</body>
</html>
As you can see, I was able to use a function as a normal value and insert it into my calculations (in the example above, I multiplied the result of the function by 2).
Arrow Function
This class of functions allows you to define functions more concisely:
// Example in ES5
var x = function(x, y) {
return x * y;
}
// Example in ES6
const x = (x, y) => x * y;
[Hive: @albro]
https://inleo.io/threads/albro/re-leothreads-snaqytc
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 200 comments.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Check out our last posts:
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.