Properties in JavaScript objects By albro
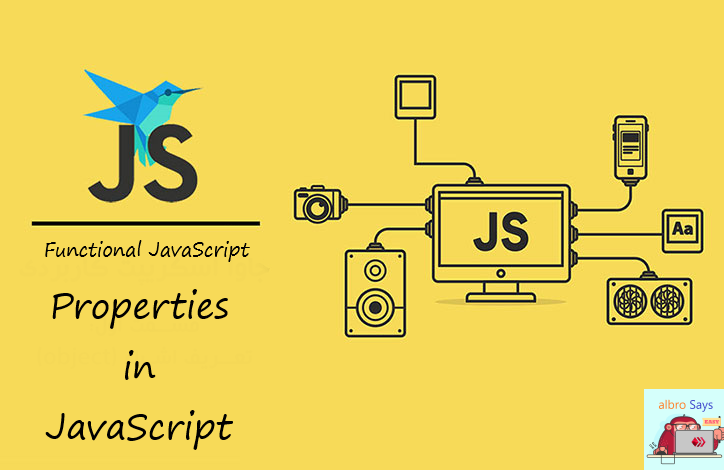
In this post, I want to talk about properties in JavaScript objects. In many ways, it can be said that properties are the most important part of an object, so I will start with them.
Properties in JavaScript objects
Properties are values given to an object, and an object can be said to be an unordered set of properties. You must know that we can remove or add or change properties, but some of them are unchangeable. Let's take a look at these.
Access properties
How to access the properties of an object in JavaScript is as follows:
objectName.property // hive.age
Or
objectName["property"] // hive["age"]
Or
objectName[expression] // x = "age"; hive[x]
Consider the following two examples.
First example:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Object Properties</h2>
<p>There are two different ways to access an object property:</p>
<p>You can use .property or ["property"].</p>
<p id="demo"></p>
<script>
var hive = {
firstname:"Hive",
lastname:"Blockchain",
age:3,
eyecolor:"red"
};
document.getElementById("demo").innerHTML = hive.firstname + " is " + hive.age + " years old.";
</script>
</body>
</html>
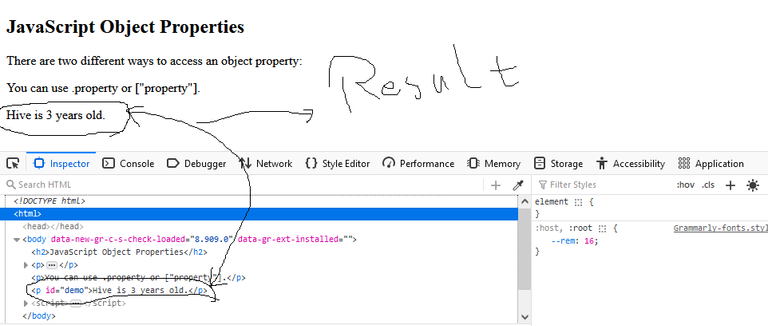
Second example:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Object Properties</h2>
<p>You can use .property or ["property"].</p>
<p id="demo"></p>
<script>
var hive = {
firstname:"Hive",
lastname:"Blockchain",
age:3,
eyecolor:"red"
};
document.getElementById("demo").innerHTML = hive["firstname"] + " is " + hive["age"] + " years old.";
</script>
</body>
</html>
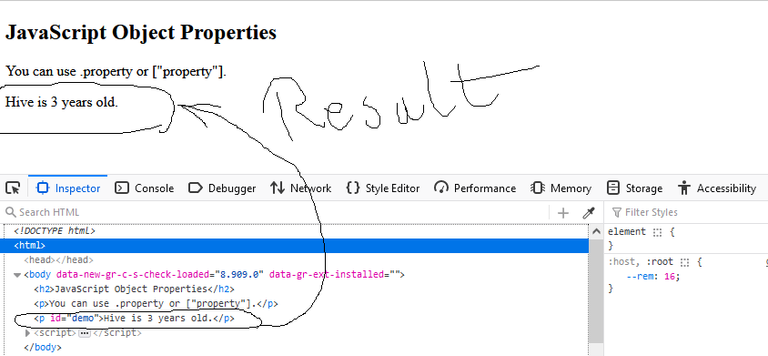
As you can see, both methods return the same result and the choice is yours which method to use.
Using the for...in
loop
The for...in
loop in JavaScript cycles through all the properties of an object and performs a specific action based on the code you wrote for it.
for (variable in object) {
// code to be executed
}
The code block placed inside the for...in
loop is executed on each object's properties. Consider the following example:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Object Properties</h2>
<p id="demo"></p>
<script>
var txt = "";
var platform = {fname:"Hive", lname:"Blockchain", age:3};
var x;
for (x in platform) {
txt += platform[x] + " ";
}
document.getElementById("demo").innerHTML = txt;
</script>
</body>
</html>
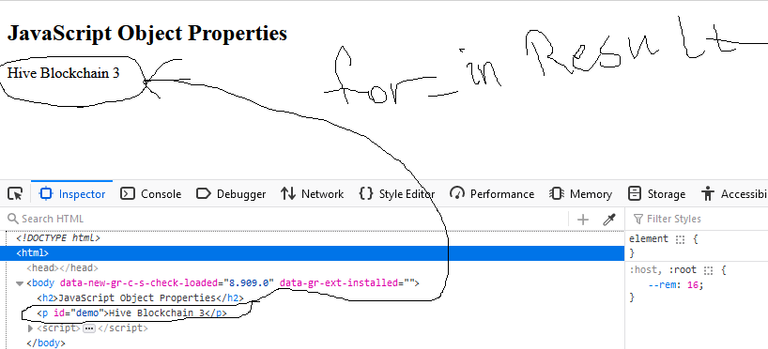
Add new properties
To add new properties, just use the same property access rule. Example:
var platform = {
firstname:"Hive",
lastname:"Blockchain",
age:3,
eyecolor:"red"
};
platform.nationality = "English";
document.getElementById("demo").innerHTML =
platform.firstname + " is " + platform.nationality + ".";
</script>
The output of this code will be "Hive is English.".
Note: You are not allowed to use reserved words to name properties of objects. The rules for naming variables are also true in the field of object properties. Reserved words in programming languages are special words that have been reserved by their developers for special purposes. For example, the word function
is a reserved word that is used to define functions. You cannot name your variables function
. Of course, you can add something to it; For example, myFucntion
is fully accepted.
Remove properties
The delete
keyword can remove a specific attribute from an object. Consider the following example:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Object Properties</h2>
<p>You can delete object properties.</p>
<p id="demo"></p>
<script>
var platform = {
firstname:"Hive",
lastname:"Blockchain",
age:3,
eyecolor:"red"
};
delete platform.age;
document.getElementById("demo").innerHTML =
platform.firstname + " is " + platform.age + " years old.";
</script>
</body>
</html>
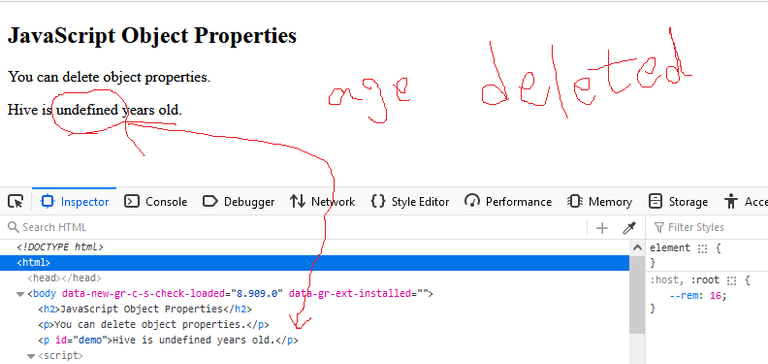
As you can see, the output of this code is "Hive is undefined years old.
", which means that Hive's age
has been removed from the object and now the value of undefined
has been returned to us.
A few tips about the delete
keyword:
- This keyword removes both the attribute and the value of that attribute.
-
After using
delete
, you can no longer access that feature unless you create it again. - This keyword is made to use properties of objects and has no effect on functions or variables.
- You should not use this keyword on default properties defined by JavaScript itself. If you do so, your program may stop altogether.
What is Attribute?
All properties have a name and a value. Now this name is considered an attribute for that property. Other attributes include enumerable
, configurable
, and writable
. In fact, the job of these attributes is to determine how a feature can be accessed. For example, is it readable? Is it also writable?
https://inleo.io/threads/albro/re-leothreads-czvypwew
The rewards earned on this comment will go directly to the people ( albro ) sharing the post on LeoThreads,LikeTu,dBuzz.
Congratulations!
✅ Good job. Your post has been appreciated and has received support from CHESS BROTHERS ♔ 💪
♟ We invite you to use our hashtag #chessbrothers and learn more about us.
♟♟ You can also reach us on our Discord server and promote your posts there.
♟♟♟ Consider joining our curation trail so we work as a team and you get rewards automatically.
♞♟ Check out our @chessbrotherspro account to learn about the curation process carried out daily by our team.
🥇 If you want to earn profits with your HP delegation and support our project, we invite you to join the Master Investor plan. Here you can learn how to do it.
Kindly
The CHESS BROTHERS team
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.