Constructor in JavaScript By albro
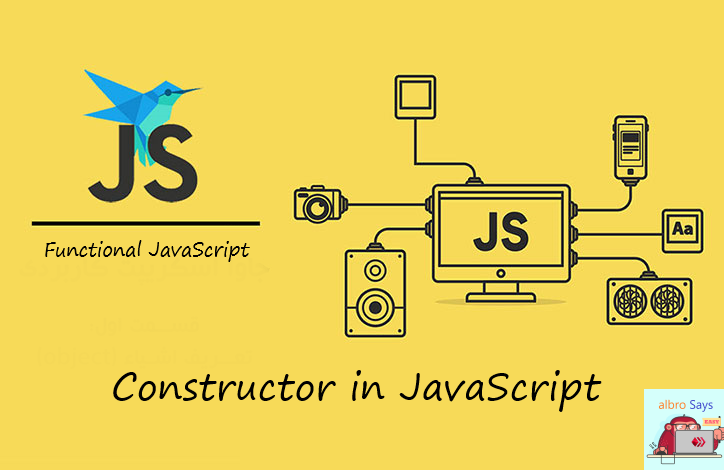
Today I want to talk about constructor in JavaScript and how they work. This topic is one of the main topics about objects in any programming language so don't miss this post.
What is Constructor in JavaScript?
What does the constructor build? All the examples I gave in the previous sections of JavaScript objects were limited, because they only created one object, and if I wanted to create several objects, I had to do it one by one and manually. This method is not an optimal method and sometimes I need to have a general design so that I can make several objects from it at the same time (like an industrial mold used in factories). To do this, I need constructors, which are actually a function.
Constructors are functions that determine the outline and structure of objects (build), so the objects created with a constructor will be of the same type.
Take a look at the following example:
function Person(username, rc, age, eye) {
this.username = username;
this.rc = rc;
this.age = age;
this.eyeColor = eye;
}
The above function is a constructor.
Note: One of the recommended ways to name constructors is to capitalize their first letter.
To create objects of the same type, you must call the constructor function with the new
keyword:
var myFriends = new Person("ocdb", 100, 5, "blue");
var myLove = new Person("albro", 100, 1, "green");
You can see that I used the constructor I defined above to create new objects called myFriends
and myLove
.
The this keyword in constructors
The this
keyword refers to the object that owns the code. For example, if I use this
inside an object, it refers to the object itself.
But this keyword has no value in constructors and is only a temporary replacement for the new object. In other words, when a new object is created from the constructor, the value of this
becomes the same as the new object, but before that, it has no value.
Note: Remember that this
is not a variable but a keyword, so you cannot change its value yourself.
Add methods and properties to the constructor
Adding a property to a specific object is very easy:
myFriends.nationality = "English";
But if I do this, this property is only added to myFriends
and nothing happens to myLove
.
Also, adding methods is not so difficult, it is exactly like adding properties:
myFriends.name = function () {
return this.username+ " " + this.age;
};
Again, I know that this method only adds to myFriends
and nothing happens to myLove
.
What if I want to add this feature and method to every object that is created? Then I have to use constructors. The method of adding specific values to the constructor is not the same as the previous methods, and the following code is not correct:
Person.nationality = "English";
This doesn't add anything to your constructor, but to add a specific value to a constructor I have to add it directly to the constructor itself:
function Person(username, rc, age, eye) {
this.username= username;
this.rc = rc;
this.age = age;
this.eyeColor = eyecolor;
this.nationality = "English";
}
In this way, every object created with this constructor will have a default value (i.e. "English
").
Adding methods is the same way:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Object Constructors</h2>
<p id="demo"></p>
<script>
// Constructor
function Person(username, rc, age, eye) {
this.username = username;
this.rc = rc;
this.age = age;
this.eyeColor = eye;
this.state = function() {
return this.username + " Have " + this.rc + " RC"
};
}
// Making an object from Person
var myFriends = new Person("ocdb", 100, 5, "blue");
// Show state
document.getElementById("demo").innerHTML =
myFriends.state();
</script>
</body>
</html>
The output of this code will be "ocdb Have 100 RC
".
I will give another example. Suppose I want to change the name of an individual, then I can add a method to the constructor that does this for me:
function Person(username, rc, age, eye) {
this.username = username;
this.rc = rc;
this.age = age;
this.eyeColor = eyeColor;
this.changeRC = function (val) {
this.rc = val;
};
}
In this code, the changeRC()
function adds the value you give as val
to the rc
attribute. For example, if we make something for my myLove
:
var myLove = new Person("leo.voter",100,48,"green");
I can change the rc
value (the current value is 100) like this:
myMother.changeRC(60);
Default constructors in JavaScript language
JavaScript has many constructors by default. Look at the following examples:
<!DOCTYPE html>
<html>
<body>
<h2>JavaScript Object Constructors</h2>
<p id="demo"></p>
<script>
var x1 = new Object(); // A new Object object
var x2 = new String(); // A new String object
var x3 = new Number(); // A new Number object
var x4 = new Boolean(); // A new Boolean object
var x5 = new Array(); // A new Array object
var x6 = new RegExp(); // A new RegExp object
var x7 = new Function(); // A new Function object
var x8 = new Date(); // A new Date object
// Display the type of all objects
document.getElementById("demo").innerHTML =
"x1: " + typeof x1 + "<br>" +
"x2: " + typeof x2 + "<br>" +
"x3: " + typeof x3 + "<br>" +
"x4: " + typeof x4 + "<br>" +
"x5: " + typeof x5 + "<br>" +
"x6: " + typeof x6 + "<br>" +
"x7: " + typeof x7 + "<br>" +
"x8: " + typeof x8 + "<br>";
</script>
<p>There is no need to use String(), Number(), Boolean(), Array(), and RegExp()</p>
</body>
</html>
Of course, the Math
object does not exist in the above example because it is a global object and you cannot use new
on it. Also, never use object for initial data, I have given the above example only for practice and otherwise it is not useful. So:
-
Use
{}
instead ofnew Object()
. -
Use
""
instead ofnew String()
. -
Use
12345
instead of newNumber()
. -
Use
true / false
instead ofnew Boolean()
. -
Use
[]
instead ofnew Array()
. -
Use
/()/
instead ofnew RegExp()
. -
Use
function(){}
instead ofnew Function()
.
Congratulations @albro! You have completed the following achievement on the Hive blockchain And have been rewarded with New badge(s)
Your next target is to reach 80 posts.
You can view your badges on your board and compare yourself to others in the Ranking
If you no longer want to receive notifications, reply to this comment with the word
STOP
To support your work, I also upvoted your post!
Check out our last posts:
Thanks for your contribution to the STEMsocial community. Feel free to join us on discord to get to know the rest of us!
Please consider delegating to the @stemsocial account (85% of the curation rewards are returned).
You may also include @stemsocial as a beneficiary of the rewards of this post to get a stronger support.